React is renowned for its efficiency and performance optimizations. Two critical tools for achieving this are useMemo and useCallback, which play a pivotal role in optimizing React components by memoizing values and functions.
Let’s take a closer look to understand what makes useMemo and useCallback necessary, why we use them, how they do their jobs, and what makes useMemo different from useCallback.
What is memoization?
Memoization is a technique used in programming to optimize functions by caching their results. It is often used to improve the performance of functions that are computationally expensive by storing the results of function calls and returning the cached result when the same inputs are provided again. In JavaScript, this technique is commonly employed to speed up recursive or repetitive calculations.
It can be particularly useful in situations where the same function is called multiple times with the same input arguments. By caching the results of the function, you can avoid redundant computation and significantly improve the performance of your code.
Memoization is like a magic trick for computers. It helps them remember answers to questions they’ve already figured out so they don’t have to work really hard to figure it out all over again.
What is the need for useMemo & useCallback ?
React primarily focuses on ensuring that our user interface (UI) stays synchronized with the state of our application. It achieves this by continuously monitoring the changes in our code and efficiently updating the UI. This is accomplished by comparing the Virtual DOM to the Real DOM and only re-rendering when necessary.
With each re-render, React instructs how the DOM should appear, depending on the current state of our application. In general, React is well-optimized, and re-rendering is not a performance issue. However, there are scenarios where the Real DOM might not update promptly or it takes time to identify the differences between the Real DOM and Virtual DOM. This can result in performance issues, such as the UI not reflecting changes quickly after a user action.
Learn more about Virtual DOM and it’s working here.
In such cases, we can use tools like useMemo and useCallback to enhance the performance of our React application.
These optimizations work by:
- Minimizing the amount of work required during a specific render.
- Reducing the frequency of component re-renders.
Understanding the useMemo Hook
useMemo is a React hook designed to memoize the result of a function and cache the result of a calculation between re-renders.
How does useMemo work
const cachedValue = useMemo(calculateValue, dependencies)
Here’s how it works:
- On the initial render, useMemo returns the result of calling the calculateValue function with no arguments.
- In subsequent renders, it behaves differently. If the dependencies array has not changed, useMemo will return the cached result from the last render, without re-invoking calculateValue. This is an optimization to avoid unnecessary recalculations.
In practical terms, this means that the calculateValue function is only invoked when any of the dependencies in the dependencies array have changed. If none of the dependencies have changed, the previously memoized result is retrieved.
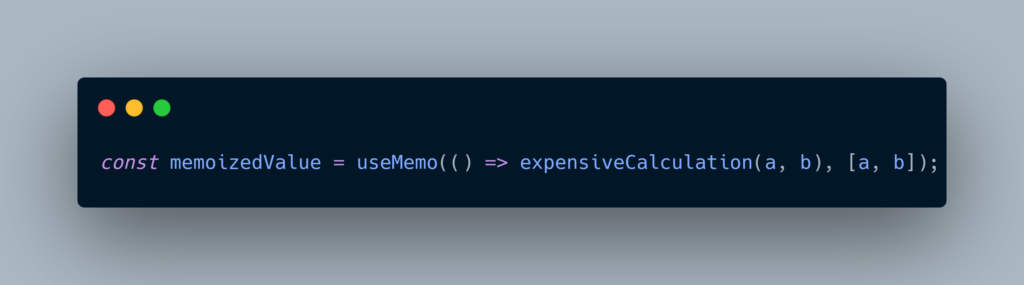
Example: expensiveCalculation is only invoked when either a
or b
changes. Otherwise, the previously memoized result is retrieved, effectively avoiding unnecessary recomputation.
When to use useMemo
- Utilize useMemo when you need to memoize the outcome of an expensive calculation or the result of the function.
- It is most effective for caching values that don’t change frequently, such as the component’s props.
Learn more about Props and State here.
Understanding the useCallback hook
useCallback is a hook that lets you cache a function definition between re-renders and is particularly beneficial when you need to pass functions down to child components and want to minimize re-renders. The Reactย useCallbackย Hook returns a memoized callback function.
Learn more about React Hooks here.
How does useCallback work?
const cachedFn = useCallback(fn, dependencies)
- When you call useCallback, you provide it with a function (fn) that you want to memoize and an array of dependencies (dependencies).
- On the initial render, useCallback creates and returns a memoized version of the function (cachedFn).
- On subsequent renders, useCallback compares the current dependencies with the previous ones. If the dependencies have not changed, it returns the same cached function reference (cachedFn) from the previous render without re-creating the function.
- If any of the dependencies in the array change, useCallback re-evaluates the function and returns a new memoized version, ensuring that the function remains up-to-date when its dependencies change.
In practical terms, this means that you can use useCallback to maintain a consistent function reference, which is especially useful when passing functions as props to child components. By preventing unnecessary function re-creation, it can help minimize re-renders in your React application, resulting in better performance.
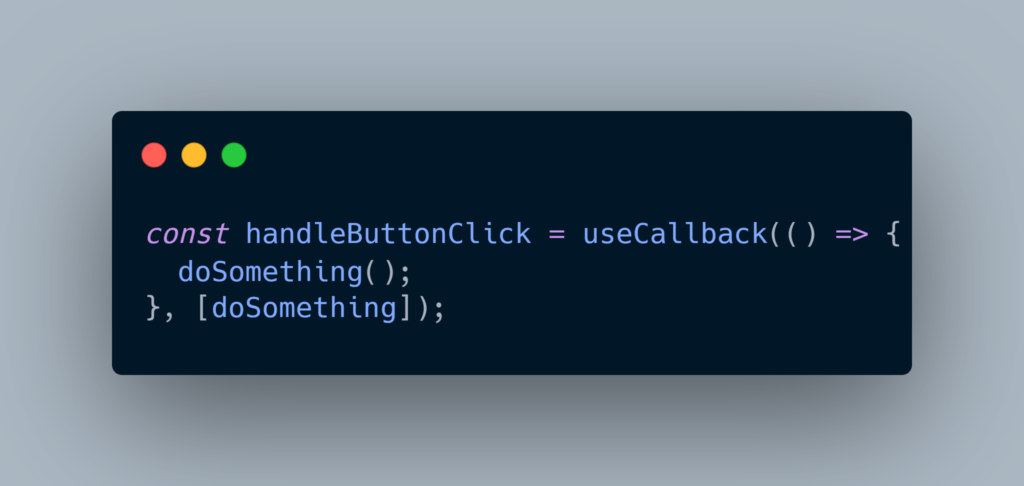
Example: handleButtonClick remains the same as long as doSomething remains constant. This is beneficial when you need to ensure that the reference to the function does not change, preventing superfluous renders in child components.
When to use useCallback( )
- Apply useCallback when you need to memoize functions or callback handlers, especially when passing functions to child components.
- This hook is ideal when the function’s reference must remain constant to prevent unnecessary re-renders.
Key differences
- Use Case: The primary distinction between useMemo and useCallback lies in their intended use. useMemo is for memoizing values, while useCallback is for memoizing functions or callback handlers.
- Dependencies: useMemo requires an array of dependencies as its second argument, recalculating the memoized value when any of these dependencies change. On the other hand, useCallback uses a function as its first argument and an array of dependencies as its second argument, focusing on memoizing the function itself rather than its result.
- Performance Implications: Both hooks contribute to performance optimization, but incorrect usage can have consequences. Overuse of useCallback may lead to excessive memory consumption because it retains function instances.
Conclusion
In conclusion, useMemo and useCallback are essential tools in React for making things faster.
useMemo helps by saving the result of a function, so it only recalculates when necessary. This can make your app run faster.
useCallback does a similar thing, but it’s for functions. It makes sure the same function is used when it’s needed, preventing extra work and making your app more efficient.
These tools are handy for optimizing your React app.
References: