Introduction to React
Welcome to the dazzling world of React! It’s a fantastic, free, and open-source JavaScript library that makes building stunning user interfaces a breeze. With React, you can create all sorts of web goodies, from single-page wonders to mobile magic.
It lets you bring your creative ideas to life. You can build stunning websites and apps that are both visually appealing and functional, giving you the opportunity to make your mark in the fast-paced online world.
So, get ready to embark on an exciting journey into web development. React is here to make your life easier and your projects more awesome. Let’s dive in and discover the magic of React together!
Prerequisites of React.js
Before you start, make sure you have these essential prerequisites in place:
- Solid JavaScript Foundation: Develop a good understanding of JavaScript fundamentals, including variables, functions, arrays, and objects. It’s the language that powers React!
- HTML Proficiency: Familiarize yourself with HTML, the building blocks of web pages. Learn about tags, elements, and document structure to create dynamic interfaces with React.
- CSS Basics: Get comfortable with CSS to style your React components. Learn about selectors, properties, and layouts to make your UI visually appealing.
- Node.js Knowledge: Install and understand Node.js, a JavaScript runtime environment. Node.js enables you to leverage npm (Node Package Manager) for managing dependencies in your React projects.
- Command Line Familiarity: Gain confidence in using the command line interface. Navigate directories, run commands, and execute scripts to manage and deploy your React applications.
- Code Editor Mastery: Choose a code editor that suits your workflow. Popular options like Visual Studio Code, Sublime Text, or Atom provide powerful tools for writing React code efficiently.
- Curiosity and Persistence: Cultivate a mindset of curiosity and eagerness to learn. React is a dynamic ecosystem, so be prepared to explore new concepts, adapt to changes, and persevere through challenges.
With these prerequisites in place, you’re well-equipped to start your React.js journey. Enjoy the process of building amazing user interfaces and creating engaging web experiences!
Setting Up a React Development Environment
Create React App
Most popular & recommended way to set up a new React project. It provides a preconfigured development environment with all the necessary tools and dependencies
npx create-react-app [name of application]
Manual Configuration
You can manually set up a React project for more flexibility and customisation. This involves manually configuring tools like Babel and Webpack.
Vite
Vite is a rapid development tool that offers a fast and efficient development experience specifically optimized for modern web projects.
>> npm create vite@latest
>> cd project directory
>> npm install
Mastering React Project Structure: A Comprehensive Guide to Essential Files and Directories
One of the most common and recommended ways to structure a react project is by following Single Responsibility Principle meaning that every component should have only one clear duty. Read more about it here.
๐ project-name
โโโ ๐ public
โ โโโ ๐ index.html
โ โโโ ๐ assets
โ โโโ ๐ผ๏ธ logo.png
โ โโโ ๐ styles.css
โโโ ๐ src
โ โโโ ๐ index.js
โ โโโ ๐ App.js
โ โโโ ๐ components
โ โ โโโ ๐ Header.js
โ โ โโโ ๐ Sidebar.js
โ โ โโโ ๐ Footer.js
โ โโโ ๐ pages
โ โ โโโ ๐ Home.js
โ โ โโโ ๐ About.js
โ โ โโโ ๐ Contact.js
โ โโโ ๐ styles
โ โ โโโ ๐ main.css
โ โ โโโ ๐ header.css
โ โ โโโ ๐ footer.css
โ โโโ ๐ utils
โ โ โโโ ๐ api.js
โ โ โโโ ๐ helper.js
โ โ โโโ ๐ validation.js
โ โโโ ๐ assets
โ โโโ ๐ผ๏ธ images
โ โ โโโ ๐ผ๏ธ image1.png
โ โ โโโ ๐ผ๏ธ image2.png
โ โโโ ๐ fonts
โ โโโ ๐ font1.ttf
โ โโโ ๐ font2.ttf
โโโ ๐ .gitignore
โโโ ๐ package.json
โโโ ๐ package-lock.json
โโโ ๐ babel.config.js
โโโ ๐ webpack.config.js
For more details and an in-depth guide to different approaches, Read More.
Features of React
Let’s learn more about the awesome features of React
- Follows Component-Based Architecture
- Effortless One-Way Data Binding
- Follows Reconciliation Algorithm
- Component Lifecycle Methods: A Series of Hooks & Methods
- JSX: The Expressive Web Development Language
- Declarative Syntax: Simplifying Web Development
- Virtual DOM: Efficient UI Rendering
Component-Based Architecture
React follows a component-based approach, allowing developers to break down the user interface into reusable and self-contained components. This modular structure promotes code reusability and simplifies the development process.

In React, components are your secret weapons! They are like puzzle pieces that fit together to create stunning web interfaces.
Here’s why they’re so awesome:
- Reusability: Components can be reused throughout your project, saving you time and effort.
- Modularity: Each component is self-contained, making it easier to manage and maintain.
- Building Blocks: Think of components as LEGO bricks that you can assemble to create something amazing!
One-Way Data Binding
React follows a unidirectional data flow, where data flows from parent components to child components. This one-way data binding simplifies the debugging process and ensures predictable data flow within the application.
The child component can read the data but can’t update it directly. So, the child component emits an event to the parent and the parent component updates it.
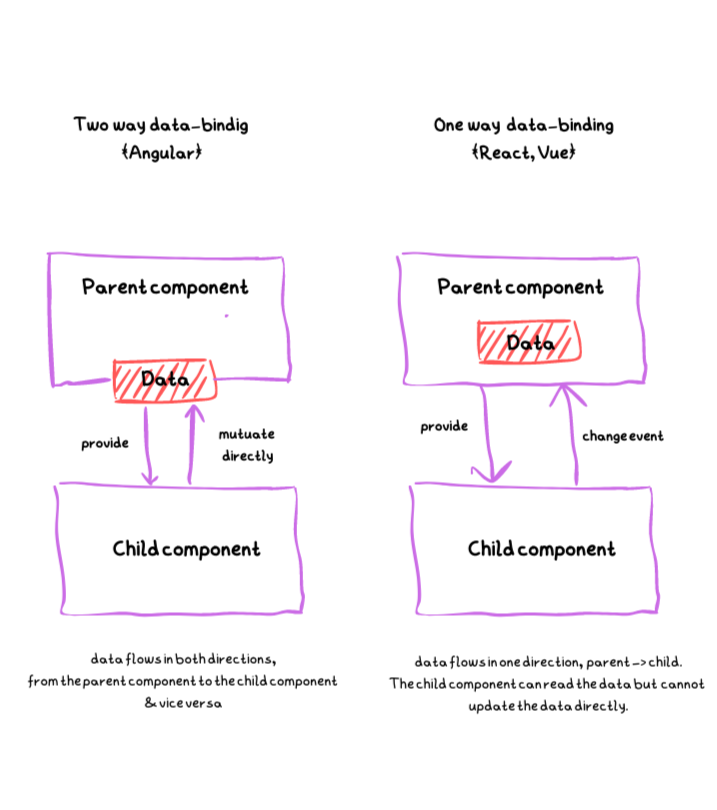
Wave goodbye to tangled data webs! React’s unidirectional data flow ensures a clear and predictable flow of data, making debugging and understanding your application a breeze.
Reconciliation Algorithm

React’s reconciliation algorithm is like a peacekeeper between the Virtual DOM and the actual DOM. It efficiently determines the minimal changes needed to update the UI, further boosting performance.
Virtual DOM
React utilizes a virtual DOM, which is a lightweight copy of the actual DOM. With the help of a virtual DOM, React efficiently manages and updates the user interface, minimizing the number of direct manipulations to the real DOM. This results in improved performance and a smoother user experience.
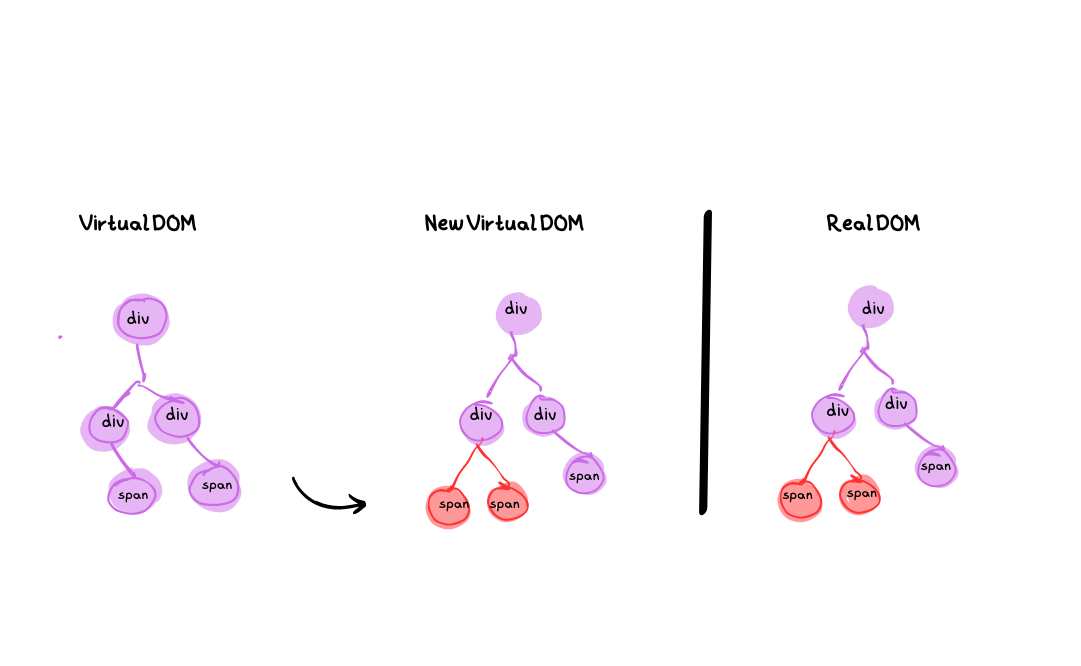
React’s Virtual DOM is like having a speedster on your team! It efficiently updates and renders only the necessary parts of the user interface, reducing page reloads and enhancing overall performance.
Learn more about Virtual DOM & its working here.
Component Lifecycle Methods
From birth to death, React provides a series of hooks and methods that allow you to control and interact with your components at different stages. Whether you want to initialize state, fetch data, or clean up resources, React’s lifecycle methods offer a seamless and intuitive way to manage your component’s behaviour. With React, you’ll have the power to create dynamic and interactive web applications that respond to your users’ every move.
JSX (JavaScript XML)
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. This unique feature enables the seamless integration of HTML markup and JavaScript logic, making it easier to build dynamic and interactive UI components.
Declarative Syntax
React uses a declarative syntax, where developers describe how the user interface should look based on the application state. This declarative approach makes the code more intuitive and easier to understand, as developers focus on the desired outcome rather than manually manipulating the DOM.
You describe what you want your user interface to look like, and React takes care of the rest. Say goodbye to manually manipulating the DOM and welcome a more intuitive and efficient way of building web applications.
React’s declarative syntax empowers you to focus on the “what” instead of the “how,” making your code easier to understand, maintain, and evolve.
Got anything to say? I’d love to hear from you. Contact me.