Why is it important to Structure React Project?
Having a well-organized project structure is essential for success in React. It’s crucial to structure React files properly to create applications that are easy to maintain, scalable, and reusable as it improves readability, maintainability, and scalability while promoting separation of concerns and code reuse.
By understanding how each element contributes to a solid framework, you’ll be equipped to build maintainable and scalable React projects. Let’s delve into the details and uncover the strategies for crafting exceptional applications that excel in the digital realm!
Guidelines to Create a Clean & Maintainable React Project Structure
When it comes to structuring a React project, there isn’t a one-size-fits-all approach that works for everyone. However, making thoughtful decisions about your codebase organization is crucial for productivity, collaboration, and long-term code maintenance. A well-structured codebase benefits both the current and future development teams. Some recommended best practices for structuring your React project are:
- Grouping by Feature or Module
- Separate Components Based on Duties
- Centralize Global Assets
- Utilize a Styles Directory
- Use a Configuration Folder
Grouping by Feature or Module
One effective approach is to group related components, styles, and utility functions together based on their feature or module. By organizing your project in this way, you can easily locate and manage code related to specific features. This modular structure promotes reusability and allows for better separation of concerns.
Separate Components Based on Duties
To maintain a clear separation of responsibilities, it’s helpful to differentiate between presentational components and container components. Each React component should only be responsible for one task and should not perform functionality that is not related to its responsibility, this principle is known as Single Responsibility Principle and is considered one of the optimal choices to structure React project.
Centralize Global Assets
Consider creating dedicated directories to store global assets such as fonts, images, and icons. This centralization ensures easy access to these assets throughout your project and simplifies maintenance. Additionally, it prevents asset duplication and promotes consistency in your application’s visual elements.
Utilize a Styles Directory
To manage your styles effectively, create a directory specifically for stylesheets. You can include global styles as well as styles that are specific to individual components or modules. Consider using CSS modules, CSS-in-JS libraries, or preprocessors like Sass to encapsulate styles and prevent global namespace collisions. This organization enables better style reusability and helps you maintain a consistent look and feel across your application.
Use a Configuration Folder
To keep your project root clean and maintain a clear separation of concerns, consider creating a dedicated directory for configuration files. This is where you can store essential files like Babel and Webpack configurations. By isolating these files, you can focus on the core development tasks while keeping your project’s configuration easily accessible.
Project structure and key files
When you organize your codebase effectively, you lay a strong foundation for a robust React application, making development, maintenance, and collaboration simpler. Let’s understand the roles and responsibilities of different files and directories in a react project structure.
src:
This directory is where the magic happens! It’s like the beating heart of your React project, where you’ll find the majority of your code.
- index.js: This file acts as the entry point for your React application. It’s responsible for rendering the root component and attaching it to the DOM.
- App.js: This file represents the main component of your application and handles the overall layout, routing, and logic, rendering other components as needed.
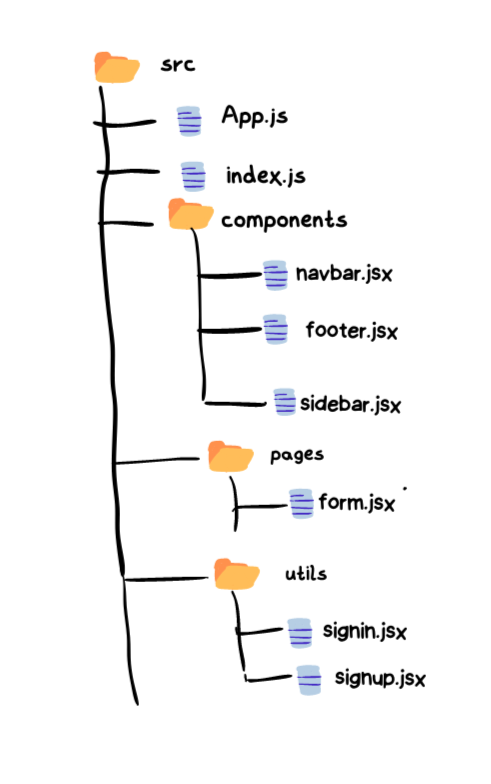
- components: This is where you’ll discover reusable components. These are like building blocks that you can use throughout your app, saving you time and effort.
- pages: Ah, the pages directory. It’s like a collection of different scenes in a movie. Each component here represents an individual page or view in your application, creating a seamless user experience.
- styles: Time to get stylish! In this directory, you can find all the CSS or other styling files that give your components their unique look and feel. It’s like your fashion closet for your app.
- utils: Who doesn’t love some handy helpers? In the utils directory, you can store utility functions or modules that provide extra superpowers to your app. They make your life easier, just like having a sidekick.
- assets: It stores the static goodiesโimages, icons, and fonts that bring visual life to your app.
public:
This directory is home to the static assets and files that stay as they are during the build process.
- index.html: This HTML template serves as the foundation for your React application and generally includes a root DOM element where your React components are mounted.
node_modules:
Okay, let’s talk dependencies. This directory is like the VIP area where all the dependencies you installed for your project hang out. It’s automatically managed by the package manager (npm or Yarn), so you can focus on creating cool stuff.
package.json
pacakge.json keeps track of your project’s metadata, dependencies, and scripts. We can define configurations and dependencies here.
package-lock.json or yarn.lock
These lock files are generated by the package manager to ensure consistent and reproducible installations of dependencies. These lock files make sure your dependencies are installed consistently and reliably. They’re like the bodyguards of your project, keeping things secure and stable.
babel.config.js or .babelrc
These files store the configuration for Babel, which determines how JavaScript code should be transpiled to ensure compatibility with different browsers.
webpack.config.js
This file contains the configuration for Webpack, which handles bundling and building your React project. It defines entry points, output paths, and various loaders and plugins.
.gitignore:
This particular file acts as a trusted confidant, providing instructions to Git version control on which files and directories should be disregarded. Its purpose is to inform Git about what should be kept confidential, including build artifacts, dependencies, and any sensitive information. Think of it as your personal vault, ensuring the protection of valuable data.
React Project Structure: Dos and Don’ts

DO’s
- Break down your application into reusable and self-contained components
- Follow Single Responsibility Principle
- Use meaningful names for directories, files, and components
- Organize your files based on their functionality, grouping related components, styles, and utilities in their respective directories. This logical grouping streamlines navigation and reduces the risk of misplaced files.
- Distinguish between container components that handle logic and data management and presentational components responsible for rendering UI elements
DON’T’S
- Avoid deep nesting of directories as it can lead to confusion and make it challenging to locate files.
- Don’t mix unrelated functionalities
- Don’t use vague or generic names for your components or directories
- Avoid mixing unrelated components or styles to prevent code clutter and potential conflicts.
So there you have it, the lowdown on the core files and directories you’ll encounter in a React project. Keep in mind that the structure might vary based on the complexity of your project, specific libraries or frameworks you use (like Redux or Next.js), and your personal style. Just remember, a well-organized structure is the key to making your React codebase readable, maintainable, and ready to conquer the world!